Circle
Categories: generativepy generative art
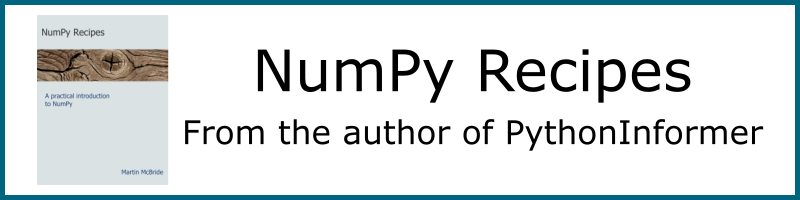
The Circle
class draws circles, arcs, sectors and segments.
There is also a circle
function that just creates a circle as a new path.
Circle class methods
The Circle
class inherits add
, fill
, stroke
, fill_stroke
, path
, clip
and other methods from Shape.
It has an additional method:
- of_center_radius
- as_arc
- as_sector
- as_segment
of_center_radius
Creates a circle based on the centre point and radius.
of_center_radius(center, radius)
Parameter | Type | Description |
---|---|---|
center | (number, number) | A tuple of two numbers, giving the (x, y) position of the centre of the circle. |
radius | number | A number, giving the radius of the circle. |
as_arc
Modifies a circle, to show only an arc. An arc is part of the circumference of the circle.
as_arc(start_angle, end_angle)
Parameter | Type | Description |
---|---|---|
start_angle | number | A number, giving the start angle of the arc |
end_angle | number | A number, giving the end angle of the arc |
This is used as a modifier with of_center_radius
, to draw just an arc. To draw an arc use:
Circle(ctx).of_center_radius((0, 0), 1).as_arc(0, 1).stroke(Color('black'), 0.1)
Angles are measured in radians. Angle zero lies along the positive x-axis, and the angle increases in the clockwise direction - note that this is opposite to the normal mathematical convention, where angle increases in the counterclockwise direction. The difference is due to the fact that y increases as you move down the image in generativepy.
If you are using a flipped coordinate system (see the setup
function in the drawing module), the angle increases in the counterclockwise direction.
Since an arc is a line, you should normally use the stroke
method to draw it. If you attempt to fill the arc, it will fill it as if it was a segment.
as_segment
Modifies a circle, to show only a segment. An segment is the part of the circle that is cut off by a chord.
as_segment(start_angle, end_angle)
This function works in a similar way to the as_arc
function, but it includes the area of the segment. You can fill or stroke the area, see the example below.
as_sector
Modifies a circle, to show only a sector. An sector is a "pizza slice", like you would use in a pie chart,.
as_sector(start_angle, end_angle)
This function works in a similar way to the as_arc
function, but it includes the area of the sector. You can fill or stroke the area, see the example below.
circle function
Adds a circle as a new path, without the need to create a Circle
object in code.
circle(ctx, center, radius)
Parameter | Type | Description |
---|---|---|
ctx | Context | The Pycairo Context to draw to |
center | (number, number) | A tuple of two numbers, giving the (x, y) position of the centre of the circle. |
radius | number | A number, giving the radius of the circle. |
The circle
function doesn't allow you to create arcs, segments or sectors.
Example
Here is some example code that draws circles using the class and the utility function. The full code can be found on github.
from generativepy.drawing import make_image, setup
from generativepy.color import Color
from generativepy.geometry import Circle, circle
'''
Create circles using the geometry module.
'''
def draw(ctx, width, height, frame_no, frame_count):
setup(ctx, width, height, width=5, background=Color(0.8))
# The circle function is a convenience function that adds a circle as a new the path.
# You can fill or stroke it as you wish.
circle(ctx, (1, 1), 0.7)
ctx.set_source_rgba(*Color(1, 0, 0))
ctx.fill()
# Circle objects can be filled, stroked, filled and stroked.
Circle(ctx).of_center_radius((2.5, 1), 0.7).fill_stroke(Color(0, 0, 1), Color(0), 0.05)
Circle(ctx).of_center_radius((4, 1), 0.7).as_arc(0, 1).stroke(Color(0, 0.5, 0), 0.05)
Circle(ctx).of_center_radius((1, 2.5), 0.7).as_sector(1, 3).stroke(Color('orange'), 0.05)
Circle(ctx).of_center_radius((2.5, 2.5), 0.7).as_sector(2, 4.5).fill(Color('cadetblue'))
Circle(ctx).of_center_radius((4, 2.5), 0.7).as_sector(2.5, 6).fill_stroke(Color('yellow'), Color('magenta'), 0.05)
Circle(ctx).of_center_radius((1, 4), 0.7).as_segment(1, 3).stroke(Color('orange'), 0.05)
Circle(ctx).of_center_radius((2.5, 4), 0.7).as_segment(2, 4.5).fill(Color('cadetblue'))
Circle(ctx).of_center_radius((4, 4), 0.7).as_segment(2.5, 6).fill_stroke(Color('yellow'), Color('magenta'), 0.05)
make_image("/tmp/geometry-circles.png", draw, 500, 500)
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest