generativepy.drawing3d module
Categories: generativepy generative art
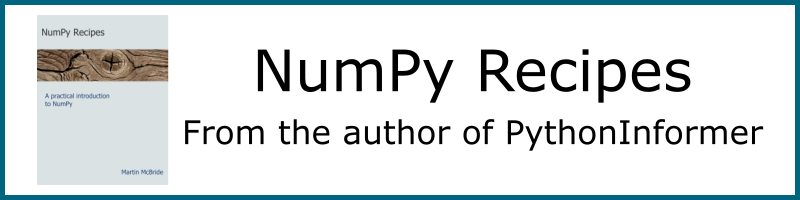
The generativepy.drawing3d module allows you to create 3d images using moderngl. It contains image creation functions, for creating PNG images, and frames.
make_3dimage
Used to create a single PNG image.
generativepy.drawing3d.make_3dimage(outfile, draw, width, height,
background=Color(0), channels=3)
Parameter | Type | Description |
---|---|---|
outfile | String | The path and filename for the output PNG file. It should end in '.png'. |
draw | Function | A drawing function object, see below. |
width | int | The width of the image that will be created, in pixels. |
height | int | The height of the image that will be created, in pixels. |
background | Color | The background colour, defaults to black. |
channels | int | The number of colour channels. 3 for RGB, 4 for RGBA |
The channels
parameter controls the transparency of the output file. To create PNGs with a transparent background, you should use RGBA.
Objects can be drawn with transparency even if RGB is used, but the image background will always be opaque unless RGBA is specified.
make_image
creates a Pycairo surface and context, then calls the user supplied draw
function to do the drawing.
The draw function must have the following signature:
draw(ctx, pixel_width, pixel_height, frame_no, frame_count)
Parameter | Type | Description |
---|---|---|
ctx | Context | A moderngl standalone context. |
pixel_width | int | The width of the image in pixels. This is the same value as the width parameter in make_image. |
pixel_height | int | The height of the image in pixels. This is the same value as the height parameter in make_image. |
frame_no | int | The number of the current frame. |
frame_count | int | The total number of frames. |
frame_no
and frame_count
only apply to functions that create a sequence of images (such as make_3dimages
below). The make_3dimage
function only ever creates one image, so the frame_no
will always be 0 and the frame_count
will always be 1.
make_3dimages
Used to create a sequence of PNG images. These can be combined into an animated GIF or video.
generativepy.drawing3d.make_3dimages(outfile, draw, width, height, count,
background=Color(0), channels=3)
Parameter | Type | Description |
---|---|---|
outfile | String | The path and base filename for the output PNG files. See below. |
draw | Function | A drawing function object, see make_image . |
width | int | The width of each image that will be created, in pixels. |
height | int | The height of each image that will be created, in pixels. |
count | int | The total number of images that will be created. |
background | Color | The background colour, defaults to black. |
channels | int | The number of colour channels. 3 for RGB, 4 for RGBA |
The outfile
parameter should be a base filepath. For example:
C:/temp/image
In this case the files will be created in the folder C:/temp, and their names will be based on the base name "image":
image00000000.png
image00000001.png
image00000002.png
etc
Once the images have been created, you can use an external program to convert them into an animated GIF or movie.
The draw function has the same signature as for make_image
. In this case though, it will be called count
times. The frame_count
will always be set to count
, and the frame_no
will increment each time draw is called. You can use the frame_no
value to draw a different image each time, to create animation.
make_3dimage_frame
Used to create a single frame.
generativepy.drawing3d.make_3dimage_frame(draw, width, height,
background=Color(0), channels=3)
Parameter | Type | Description |
---|---|---|
draw | Function | A drawing function object, see below. |
width | int | The width of the image that will be created, in pixels. |
height | int | The height of the image that will be created, in pixels. |
background | Color | The background colour, defaults to black. |
channels | int | The number of colour channels. 3 for RGB, 4 for RGBA |
This works in a similar way to make_3dimage
, but instead of saving the image to a file, it returns a frame.
make_3dimage_frames
Used to create a sequence of frames.
generativepy.drawing3d.make_3dimage_frames(draw, width, height, count,
background=Color(0), channels=3)
Parameter | Type | Description |
---|---|---|
draw | Function | A drawing function object, see make_image . |
width | int | The width of each image that will be created, in pixels. |
height | int | The height of each image that will be created, in pixels. |
count | int | The total number of images that will be created. |
background | Color | The background colour, defaults to black. |
channels | int | The number of colour channels. 3 for RGB, 4 for RGBA |
This works in a similar way to make_3dimages
, but instead of saving the images to a set of files, it returns a lazy sequence of frames.
See also
- generativepy.analytics module
- generativepy.bitmap module
- generativepy.color module
- generativepy.drawing module
- generativepy.formulas module
- generativepy.geometry module
- generativepy.geometry3d module
- generativepy.gif module
- generativepy.graph module
- generativepy.math module
- generativepy.movie module
- generativepy.nparray module
- generativepy.shape2d module
- generativepy.table module
- generativepy.tween module
- generativepy.utils module
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest