Transform
Categories: generativepy generative art
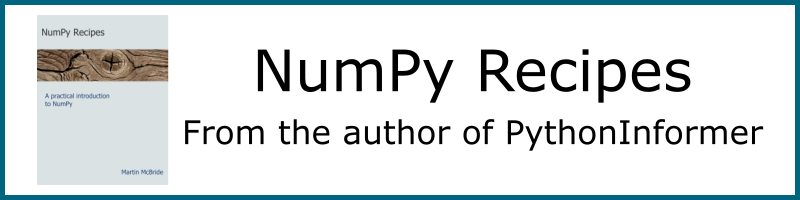
The Transform
class transforms the user space, affecting all subsequent drawing operations. Several transformations are provided:
translate
shifts user space in the x and y directions, so that object will drawn in a new position.scale
scales user space, so that objects will be drawn larger or smaller. It is possible to scale x and y by different amounts, and to centre the scaling on any point.rotate
rotates user space, so that object will be drawn rotated. It is possible to rotate the space around any point.matrix
applies a general transformation matrix. This can be used to apply any affine transformation.
Flipping and shearing can also be applied with the above operators, as described below.
Multiple transforms can be applies at the same time, for example it is possible to rotate and scale at the same time.
Transforms affect every aspect of the drawing, including:
Shape
objects.Image
objects.- Line thickness and dash pattern.
- Patterns (eg gradients).
Applying and removing transforms
The Transform
object is a context manager, intended to be used in a with
block. The with
block determines when the transform will apply. For example:
Rectangle(ctx)... # No transform applied
with Transform(ctx).scale(2, 2):
Rectangle(ctx)... # This will be scaled
with Transform(ctx).rotate(1):
Rectangle(ctx)... # This will be scaled and rotated
Rectangle(ctx)... # This will be scaled but not rotated
Rectangle(ctx)... # No transform applied
The scaling transform applies to anything that is drawn inside the first with
block. The rotation and the scaling apply to anything that is draw inside the second with
block. When we leave each with
block, it's transform no longer applies.
Transform class methods
The Transform
is not derived from Shape
, so it doesn't inherit any of its methods.
It has the following methods:
- translate
- scale
- rotate
- matrix
It also implements the ContexManager
protocol, so it can be used in a with
statement.
Constructor
The Transform
constructor accepts a context object (similar to Shape
objects):
Transform(ctx)
translate
Translates user space.
translate(tx, ty)
Parameter | Type | Description |
---|---|---|
tx | number | Translation in the x direction |
ty | number | Translation in the y direction |
This translates user space. Anything drawn in the new user space will be shifted by (tx, ty)
scale
Scales user space.
scale(sx, sy, centre=(0, 0))
Parameter | Type | Description |
---|---|---|
sx | number | Scale in the x direction |
sy | number | Scale in the y direction |
centre | tuple of 2 numbers | Centre of scaling |
This scales user space. Anything drawn in the new user space will change size by a factor of sx
in the x direction and sy
in the y direction.
centre
is the fixed point. By default it is the origin.
rotate
Rotates user space.
rotate(angle, centre=(0, 0))
Parameter | Type | Description |
---|---|---|
angle | number | Rotation angle, clockwise, in radians |
centre | tuple of 2 numbers | Centre of rotation |
This rotates user space. Anything drawn in the new user space will rotated around centre
.
centre
is the fixed point. By default it is the origin.
matrix
Applies a transformation matrix to user space.
rotate(m)
Parameter | Type | Description |
---|---|---|
m | tuple of 6 numbers | Transform matrix |
This applies a 6 element transform matrix to user space.
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest