Turtle
Categories: generativepy generative art
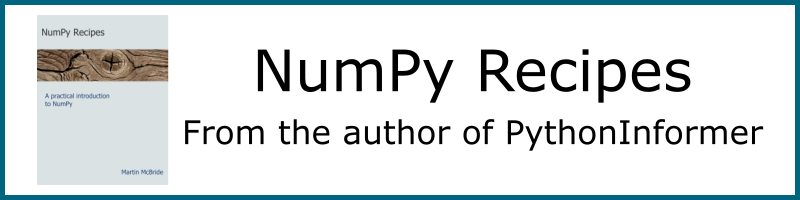
The Turtle
class implements a simple turtle graphics system.
Turtle graphics
You will probably be familiar with turtle graphics. The idea is that you have a graphics cursor that can draw lines as it moves around.
The cursor has an (x, y) position, and also a direction it is pointing in (the heading). The you can tell the turtle to move forward by a certain distance, or to turn through a certain angle to the left or right. By issuing a series of instructions, you can draw various shapes.
To allow for recursive drawing (to create fractal images) turtle graphics can push the current state onto a stack. At some later stage it can pop its previous state (position and heading) and continue from there.
The turtle can also move to different place without drawing anything.
It is possible to change the colour, thickness and dash style of the lines the turtle draws.
More than one turtle can be active at the same time, simply by creating more than one turtle object.
Turtle class methods
The Turtle
is not derived for Shape
, so it doesn't inherit any of its methods.
It has the following methods:
- forward
- left
- right
- set_heading
- move
- move_to
- set_style
- push
- pop
Constructor
The Turtle
constructor accepts a context object (similar to Shape
objects):
Turtle(ctx)
The turtle will be created with the following settings:
- Position (0, 0) is the current user space.
- Heading 0 (ie facing along the positive going x-axis).
- Line style black, width 1 unit, solid line.
forward
Moves the turtle forward, in the direction of its current heading, by the specified distance.
forward(position)
Parameter | Type | Description |
---|---|---|
distance | number | The distance to move forward. |
The turtle will move distance
units in the direction of its current heading.
It will draw a line from its current position to its new position.
Its current position will be changed to the new position.
left
Rotates the turtle heading left by angle
.
left(angle)
Parameter | Type | Description |
---|---|---|
angle | number | The angle to turn. |
The turtle will rotate its heading left by angle
. The turtle will not move, or draw anything. However, it will end up pointing is a new direction, and that will affect any forward
or move
calls.
The angle is measured in radians.
Turning left is equivalent to rotating counter-clockwise.
right
Rotates the turtle heading right by angle
.
right(angle)
Parameter | Type | Description |
---|---|---|
angle | number | The angle to turn. |
The turtle will rotate its heading right by angle
. The turtle will not move, or draw anything. However, it will end up pointing is a new direction, and that will affect any forward
or move
calls.
The angle is measured in radians.
Turning right is equivalent to rotating clockwise.
set_heading
Rotates the turtle heading to a specific angle
.
set_heading(angle)
Parameter | Type | Description |
---|---|---|
angle | number | The angle to set. |
The turtle will rotate its heading to an absolute angle
. This is equivalent to:
- Setting the heading to zero.
- Then executing
right(angle)
.
The turtle will not move, or draw anything. However, it will end up pointing is a new direction, and that will affect any forward
or move
calls.
The angle is measured in radians.
move
Moves the turtle forward, in the direction of its current heading, by the specified distance, but without drawing anything.
move(position)
Parameter | Type | Description |
---|---|---|
distance | number | The distance to move forward. |
The turtle will move distance
units in the direction of its current heading.
It will move without drawing anything. move
is exactly like forward
except that it doesn't draw anything.
Its current position will be changed to the new position.
move_to
Moves the turtle to a specific (x, y)
position, but without drawing anything.
move_to(x, y)
Parameter | Type | Description |
---|---|---|
x | number | x position |
y | number | y position |
The turtle will move to the position (x, y)
. It will not darw anything, and its crrent heading will not change.
Its current position will be changed to the new position.
set_style
Moves the turtle to a specific (x, y)
position, but without drawing anything.
set_style(color=Color(0), line_width=1, dash=None, cap=SQUARE)
Parameter | Type | Description |
---|---|---|
color | Color | line colour |
line_width | number | line width, in user units |
dash | list or None | dash pattern |
cap | cap style | line cap style |
Sets the style of the lines drawn by the turtle.
The parameters are the same as the parameters used by the stroke
method of Shape objects.
push
Moves the turtle state on a stack.
push()
The current turtle position, heading and line style will be pushed onto an internal stack. The turtle state will not be changed.
push
Retrieves the turtle state from stack.
push()
You can save the state multiple times by calling push
multiple times. Calling pop
multiple times will restore previous stored states from the newest to the oldest.
Example code
For example code see the turtle article in the tutorial.
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest