Strings
Categories: python language beginning python
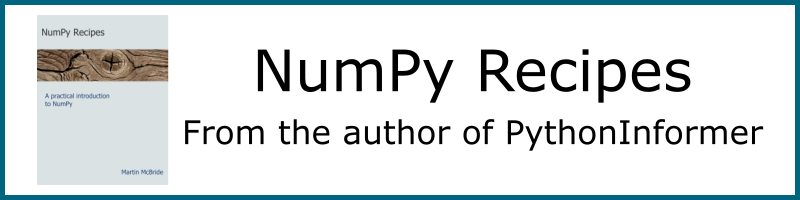
Introduction
A string is a piece of text data. Strings are used frequently in programming, for example, to display messages to the user, or to represent filenames or web URLs, and many other things.
About this lesson
In this lesson we will look at:
* Declaring strings
* The input
function
* Converting between strings and other data types
* How to use special characters in a string
Declaring strings
You can declare a string by enclosing the text in quotation marks. You can use either single quote characters ' or double quote characters ". It doesn't matter which you use, but whichever character you use to start the string you must use the same character to end it:
a = 'Hello'
b = "world"
c = 'Not valid" # Must start and end with same type
Python traditionally uses single quotes, but it doesn't matter if you prefer to use double-quotes.
The input function
The input
function provides a simple way to request user input from the console:
name = input('What is your name?')
print('Hello', name)
The input statement displays a prompt and waits for the user to type something in:
>>> What is your name?
If you type in your name and hit enter, the second line of the program (the print
statement) will display a greeting:
>>> What is your name? Martin Hello Martin
Converting between strings and other data types
You can convert almost any type of data to a string, using the str
function. Try this using the Python console:
>>> str(1 + 2) '3' >>> str(1 / 2) '0.5'
Here we calculate an integer value and convert it to a string. Then we calculate a float value and convert it to a string. In fact, str
works on almost any type of value.
You can convert numbers to strings too, using the int
and float
functions:
>>> int('5') 5 >>> float('2.3') 2.3 >>> float('abc') ValueError
If you pass in a string that cannot be converted to a number, you will get an error - a ValueError
.
Operations on strings
Python allows you to perform all sorts of functions on strings. We will meet more of these later (they will make more sense once you know an#bout lists and slices), but here are a few simple operations.
You can join two strings into one:
>>> 'Hello' + 'World' 'HelloWorld'
You can also repeat a string n
times:
>>> 'Hello' * 3 'HelloHelloHello'
There are lots of string functions, here are a selection:
>>> s = 'Hello, world!' >>> s.upper() 'HELLO, WORLD!' (converts to upper case) >>> s.center(20) ' Hello, world! ' (pads to 20 characters, with text in centre) >>> s.split() ['Hello,', 'world!'] (splits into separate words)
The last example produces a list of the separate words in the string - we will meet lists soon!
We will meet more string functions in this later in this course.
How to use special characters in a string
Sometimes you might need to include "special" characters in a string.
Quote characters
For example, what if your string contained a quote character?
s = 'This isn't easy' # ERROR
Unfortunately, this gives an error. The problem is that the ' character in isn't tells Python that the string has ended. So what Python sees is:
- the string 'This isn'
- random characters t easy' after the string, which isn't valid Python.
There are two ways to fix this:
- You can use the \ character, called the escape character, to tell Python that the following character needs special treatment. ie treat it as part of the string, not the end of the string.
- You can use enclose the string in double-quotes. In that case, a single quote character isn't anything special so gets handled normally.
u = 'This isn\'t easy'
v = "This isn't easy"
Both methods are equally valid. You can use similar methods if you need to include double quotes in a string:
w = "Using \"special\" characters"
x = 'Using "special" characters'
### The escape character \
The character \ has a special meaning - it is the escape character. But what if you need to use that actual character in your string? Well, you have to escape it. You use \\ to represent \.
s = 'How to use \\ in a string' # How to use \ in a string
Unicode characters
Python supports Unicode, which means it can handle just about every character and symbol you can imagine. That includes every character used by almost any language in the world, plus a whole variety of mathematical and scientific symbols, and lots more besides.
Normally you can just paste special characters into your Python editor direct. For example if we want to print the temperature:
t = 18
print('The temperature is', t, '° C')
If you just copy and paste that code into IDLE, the degree symbol will be copied in and will work just fine.
An alternative way, particularly if your editor doesn't support Unicode, is to use the character code. For example, the Unicode square root symbol is √. A quick web search for "Unicode square root" will locate it, and tell you that its Unicode character code is 221A (hex).
print('The square root symbol is \u221a')
The escape code \u followed by the 4 digit Unicode value adds the character to the string. Make sure you have the Unicode hex value, not the decimal value (for example square root has hex value 221a, which is equal to decimal value 8,730 - you must use the hex value).
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest