NumPy Recipes ebook
Categories: book numpy
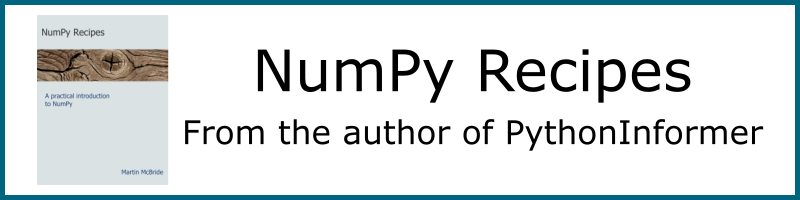
Buy now from Leanpub, Apple Books, Barnes and Noble, kobo, or other stores.
Download a free preview.
NumPy Recipes takes practical approach to the basics of NumPy
This book is primarily aimed at developers who have at least a small amount of Python experience, who wish to use the NumPy library for data analysis, machine learning, image or sound processing, or any other mathematical or scientific application. It only requires a basic understanding of Python programming.
Detailed examples show how to create arrays to optimise storage different types of information, and how to use universal functions, vectorisation, broadcasting and slicing to process data efficiently. Also contains an introduction to file i/o and data visualisation with Matplotlib.
Contents:
- 1 Introduction to NumPy
- 1.1 Installing NumPy
- 1.2 What is NumPy?
- 1.3 NumPy vs Python lists
- 1.4 Advantages of NumPy
- 1.5 NumPy universal functions
- 1.6 Compatibility with other libraries
- 2 Anatomy of a NumPy array
- 2.1 NumPy arrays compared to lists
- 2.2 Printing the characteristics of an array
- 2.3 Array rank examples
- 2.4 Data types
- 3 Creating arrays
- 3.1 Creating an array of zeroes
- 3.2 Creating other fixed content arrays
- 3.3 Choosing the data type
- 3.4 Creating multi-dimensional arrays
- 3.5 Creating like arrays
- 3.6 Creating an array from a Python list
- 3.7 Controlling the type with the array function
- 3.8 array function anti-patterns
- 3.9 Creating a value series with arange
- 3.10 Rounding error problem with arange
- 3.11 Create a sequence of a specific length with linspace
- 3.12 Making linspace more like arange using the endpoint parameter
- 3.13 Obtaining the linspace step size
- 3.14 Other sequence generators
- 3.15 Creating an identity matrix
- 3.16 Creating an eye matrix
- 3.17 Using vectorisation
- 4 Vectorisation
- 4.1 Performing simple maths on an array
- 4.2 Vectorisation with other data types
- 4.3 Vectorisation with multi-dimensional arrays
- 4.4 Expressions using two arrays
- 4.5 Expressions using two multi-dimensional arrays
- 4.6 More complex expressions
- 4.7 Using conditional operators
- 4.8 Combining conditional operators
- 5 Universal functions
- 5.1 Example universal function - sqrt
- 5.2 Example universal function of two arguments - power
- 5.3 Summary of ufuncs
- 5.4 ufunc methods
- 5.5 Optional keyword arguments for ufuncs
- 6 Indexing, slicing and broadcasting
- 6.1 Indexing an array
- 6.2 Slicing an array
- 6.3 Slices vs indexing
- 6.4 Views
- 6.5 Broadcasting
- 6.6 Broadcasting rules
- 6.7 Broadcasting a column vector
- 6.8 Broadcasting a row vector and a column vector
- 6.9 Broadcasting scalars
- 6.10 Efficient broadcasting
- 6.11 Fancy indexing
- 7 Array manipulation functions
- 7.1 Copying an array
- 7.2 Changing the type of an array
- 7.3 Changing the shape of an array
- 7.4 Splitting arrays
- 8 File input and output
- 8.1 CSV format
- 8.2 Writing CSV data
- 8.3 Reading CSV data
- 9 Using Matplotlib with NumPy
- 9.1 Installing Matplotlib
- 9.2 Plotting a histogram
- 9.3 Plotting functions
- 9.4 Plotting functions with NumPy
- 9.5 Creating a heatmap
- 10 Reference
- 10.1 Data types
More information:
If you have any comments or questions you can get in touch by any of the following methods:
- The book page on the Leanpub website.
- Signing up for the Python Informer newsletter at pythoninformer.com
- Following @pythoninformer on twitter.
- Contacting me directly by email (info@axlesoft.com).
Buy now from Leanpub, or download a free preview.
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest