Flask webserver - adding an About page
Categories: flask
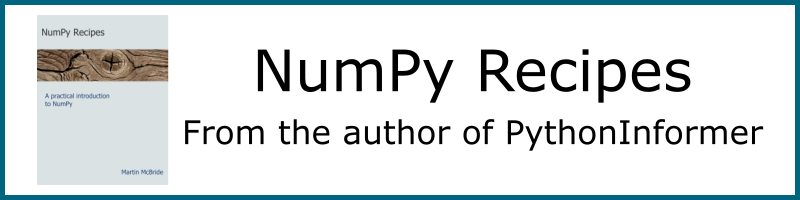
In this article we will add a second page to our website - an About page.
Using HTML templates
Our current HTML page contains the complete home page - the HTML structure plus the actual text. What we will do now is convert the HTML page into a template, containing the HTML structure but with placeholders instead of the actual text. In that way, we can reuse the same HTML file for the home page, the about page, and any other pages which follow the same layout.
Here is the updated file. Take care to get the double curly brackets exactly right:
<html>
<head>
<link rel="stylesheet" href='/static/main.css' />
</head>
<body>
<h1>{{title}}</h1>
{{content}}
</body>
</html>
As you can see, we have replaced the actual title and content text with special placeholders {{title}}
and {{content}}
.
Changing the Python code
How do we get our actual title and content to appear on the page? Well that is what render_template()
does. Change the index()
function in server.py like this:
@app.route('/')
def index():
return render_template('index.html', title='Home page',
content='My first Flask site')
As you can see, we have added two named parameters to the call, which supply values for the title and content. The render_template
function takes the index.html file and substitutes each placeholder with the value of the parameter with the same name. So:
{{title}}
becomes 'Home page'{{content}}
becomes 'My first Flask site'
Placeholders can be given any name you wish, provided you use the same name in the HTML and the function parameter. You must choose a name which is a valid Python variable name.
If you change the HTML and Python files and visit the page again in your browser, you will see that the page looks exactly the same as before - which is good.
Adding the About page
To add an About page, we need to add a new routing function to our Python code - similar to the index()
function but with different values. We will call the function about()
, because it handles the About page:
@app.route('/about')
def about():
return render_template('index.html', title='About',
content='This is the About page')
The differences between the about()
function and the index()
function are:
- The route is /about rather than /.
- The
title
andcontent
parameters have different values.
It doesn't really matter what you call you routing functions, but is is best to choose consistent and meaningful names. So in this case the About page is handled by the
about
function.
You can view the about page by visiting http://127.0.0.1/about with your browser. The home page can still be found at http://127.0.0.1
The code for this example can be found on github.
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest