Flask webserver - getting started
Categories: flask
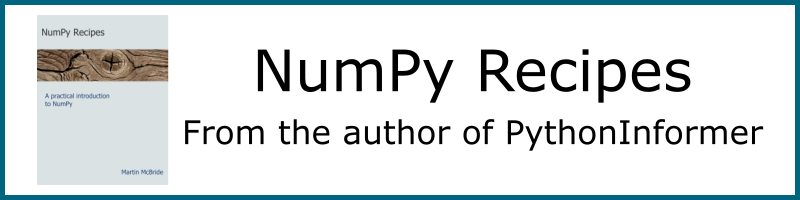
In this article we will learn how to install Flask and run up a very simple local webserver.
The code for and all other examples can be found on github in the python-libraries/flask folder.
Installing Flask
Open a command (cmd) window and use pip to install flask:
pip install flask
The code
You should first create a working folder for the project, somewhere suitable. For example c:\web-server, although you will probably want to create it where ever you keep the rest of your work.
Create a new file in your Python editor (IDLE, or whatever editor you prefer). Type in the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return 'My first Flask site'
if __name__ == '__main__':
app.run(debug=True, port=80, host='0.0.0.0')
This code can be found on github.
Save the code as server.py in your working folder. Then run the code. You should see something like this in the IDLE console:
RESTART: C:\web-server\server.py
* Running on http://0.0.0.0:80/ (Press CTRL+C to quit)
* Restarting with stat
Your web server is running!
View the page in a browser
Open a browser and type the following into the address field:
http://127.0.0.1
This is a special URL. Instead of going out on to the web to find a page, it looks for a web server on your own PC. With most browsers you can shorten this to 127.0.0.1, and it will still work. Or, instead of the numbers, you can just type in localhost if you find it easier to remember. They all do the same thing.
If all is well, you should see your message ('My first Flask site') in your browser.
What the code does
Here is the code, line by line. First we import the Flask module:
from flask import Flask
Next we create a Flask object, called app. This object runs the web server. We pass in name, the name of the module. This tells Flask where to look for the extra files we will add later - don't worry about this too much, you would only need to play with this parameter to create complex web sites.
app = Flask(__name__)
Now we get to the function index() which actually creates the web page content. In this case it is very simple, just the text My first Flask site.
The line @app.route('/'), just before the index function, is called a decorator. It tells Flask that any path which matches '/' should use the index() function to create its content. The path '/ ' is the home page of the website.
@app.route('/')
def index():
return 'My first Flask site'
The final part should be familiar, it just causes app.run if this file is executed as a python program. The parameters are set to default values which allow a browser to view our page.
if __name__ == '__main__':
app.run(debug=True, port=80, host='0.0.0.0')
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest