Creating numpy arrays with fixed values
Categories: numpy
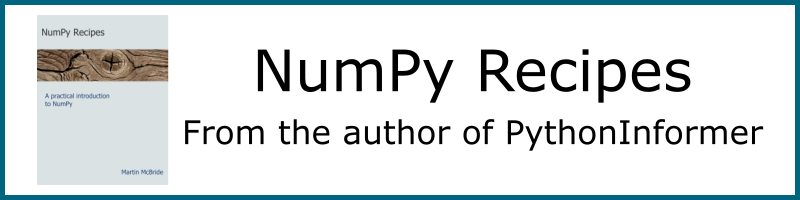
In this section we will look at how to create numpy arrays with fixed content (such as all zeros).
Here is a video covering this topic:
We will first look at the zeros
function, that creates an array full of zeros. We will use that to see how to:
- Create arrays of different shapes.
- Create arrays using different data types (such as floats and ints).
- Create like arrays (arrays that copy the shape and type of another array).
We will the look at some other fixed value functions: ones
, full
, empty
, identity
.
zeros function
The zeros
function creates a new array containing zeros. For example:
import numpy as np
a1 = np.zeros(4)
print(a1)
This will create a1
, one dimensional array of length 4. By default the array will contain data of type float64
, ie a double float (see data types).
[ 0. 0. 0. 0.]
Creating zero arrays of different shape
The shape of an array specifies the number of dimensions and the size of the array in each dimension. For example a shape of (2, 3)
specifies a 2 dimensional array consisting of 2 rows of 3 columns:
a2 = np.zeros((2, 3))
print(a2)
Here is the output:
[[ 0. 0. 0.]
[ 0. 0. 0.]]
The shape of the array is often specified by a tuple eg (2, 3)
. Of course you can use a list, or any other sequence of integers (even another numpy array if you want to).
For one dimensional arrays, you can optionally use a single integer, rather than a tuple of one item - this is what we did when we created a1
above.
Setting the data type
By default, the array is created with a data type of float64
. You can use the optional parameter dtype
to specify a different data type. For a numpy array, all the elements must be the same type.
In the code below, a2_ints
is an integer array. See the article on data types for a full list of data types:
a3 = np.zeros((2, 2), dtype=np.int32)
print(a3)
This gives:
[[0 0]
[0 0]]
zeros_like
The zeros_like
function will create a new array of zeros that is the same shape and data type as the supplied array:
b1 = np.array([[1, 2], [3, 4]])
c1 = np.zeros_like(a2_ints)
c1
now contains a new 2 by 2 array of integer zeros.
ones
The ones
function is exactly the same as zeros
, except that it fills the array with value 1. For example:
o1 = np.ones((3, 2))
print(o1)
gives:
[[1. 1.]
[1. 1.]
[1. 1.]]
You can choose the select the type, in the same way as zeros
. There is also a ones_like
function that works in an analogous way to zeros_like
.
empty
The empty
function is also similar to zeros
, except that it leaves the data array uninitialised. empty
might be slightly faster than zeros
, so it can be used if you are intending to immediately initialise the array in some other way.
Bear in mind that the uninitialised memory might not be random. It will just be whatever happens to be in the memory, so it might potentially contain user data left behind from the last time the memory was used.
There is, of course, a corresponding empty_like
function.
full
The full
function takes an extra parameter, the fill value, and uses that value to initialise the array elements:
f1 = np.full((2, 3), 7)
print(f1)
Giving:
[[ 7. 7. 7.]
[ 7. 7. 7.]]
Again, there is also a full_like
function:
b1 = np.array([[1, 2], [3, 4]])
f2 = np.full_like(b1, 3)
print(f2)
giving
[[3 3]
[3 3]]
identity
The identity
function creates an identity matrix. This is always a square, 2-dimensional array. You just need to specify the size and optional type:
i = np.identity(3)
This creates a 3 by 3 array with the diagonal elements set to 1 and everything else set to 0:
[[1. 0. 0.]
[0. 1. 0.]
[0. 0. 1.]]
You can also checkout the eye
function, which also creates a diagonal matrix, but with extra control (for example, creating a non-square array).
See also
Join the PythonInformer Newsletter
Sign up using this form to receive an email when new content is added:
Popular tags
2d arrays abstract data type alignment and angle animation arc array arrays bar chart bar style behavioural pattern bezier curve built-in function callable object chain circle classes clipping close closure cmyk colour combinations comparison operator comprehension context context manager conversion count creational pattern data science data types decorator design pattern device space dictionary drawing duck typing efficiency ellipse else encryption enumerate fill filter font font style for loop formula function function composition function plot functools game development generativepy tutorial generator geometry gif global variable gradient greyscale higher order function hsl html image image processing imagesurface immutable object in operator index inner function input installing iter iterable iterator itertools join l system lambda function latex len lerp line line plot line style linear gradient linspace list list comprehension logical operator lru_cache magic method mandelbrot mandelbrot set map marker style matplotlib monad mutability named parameter numeric python numpy object open operator optimisation optional parameter or pandas partial application path pattern permutations pie chart pil pillow polygon pong positional parameter print product programming paradigms programming techniques pure function python standard library radial gradient range recipes rectangle recursion reduce regular polygon repeat rgb rotation roundrect scaling scatter plot scipy sector segment sequence setup shape singleton slice slicing sound spirograph sprite square str stream string stroke structural pattern subpath symmetric encryption template tex text text metrics tinkerbell fractal transform translation transparency triangle truthy value tuple turtle unpacking user space vectorisation webserver website while loop zip zip_longest